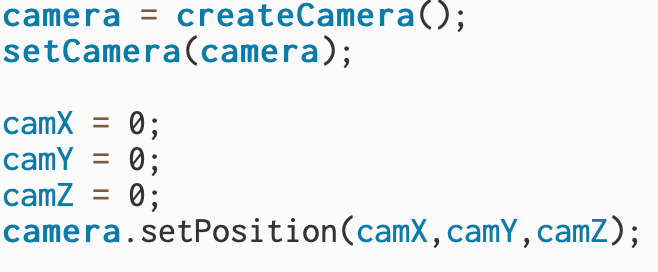
To start off, I created a camera object with variables for the x, y, and z positions.
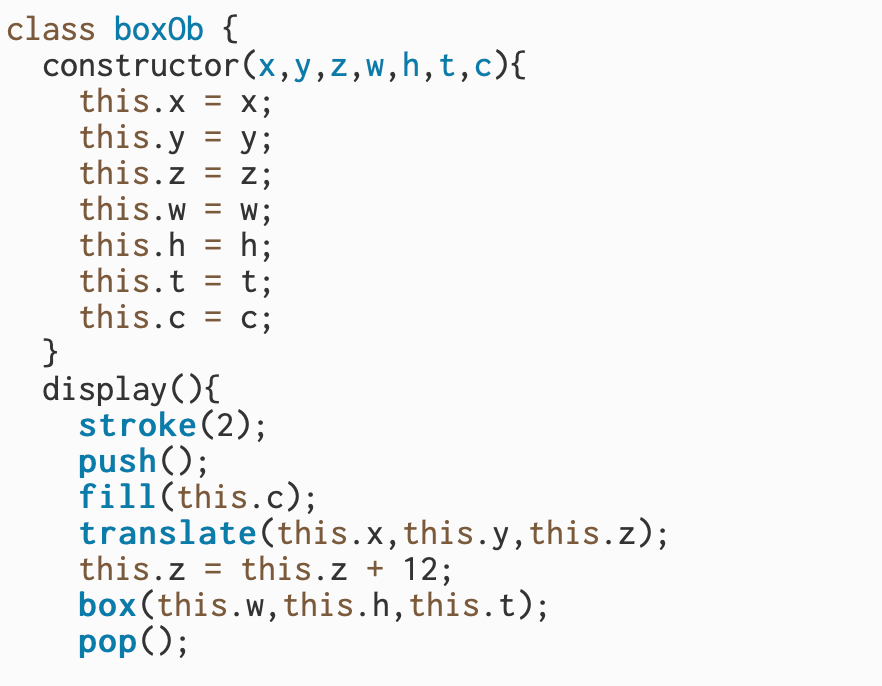
I created a box object that takes in 7 arguments (xpos, ypos, zpos, width, height, thickness/depth, and color). In the display method, I draw the box primitive with the corresponding values.
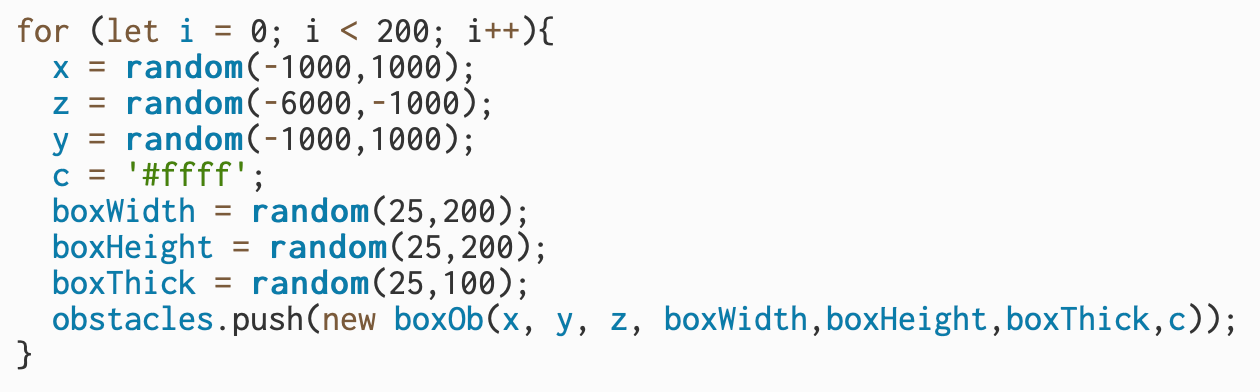
I push 200 box objects into the array called obstacles. I randomize the x,y, and z positions, and set the color to default white. I also randomize the box dimensions as well.
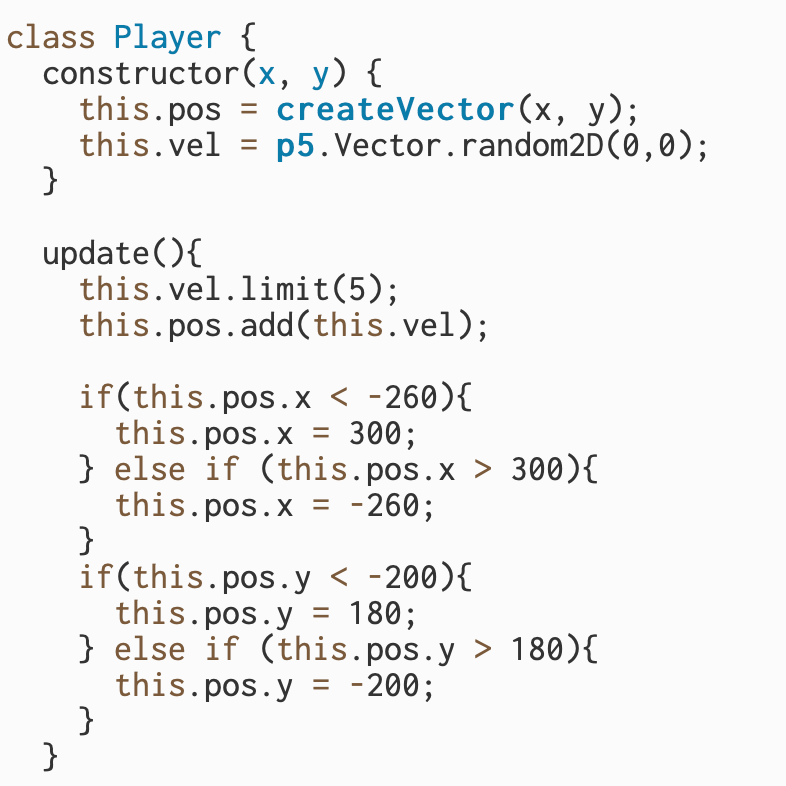
I also created a player object, and assigned a p5.Vector to it. The vector is a random2D element, because I only want the player to be able to move in the x-y dimension.
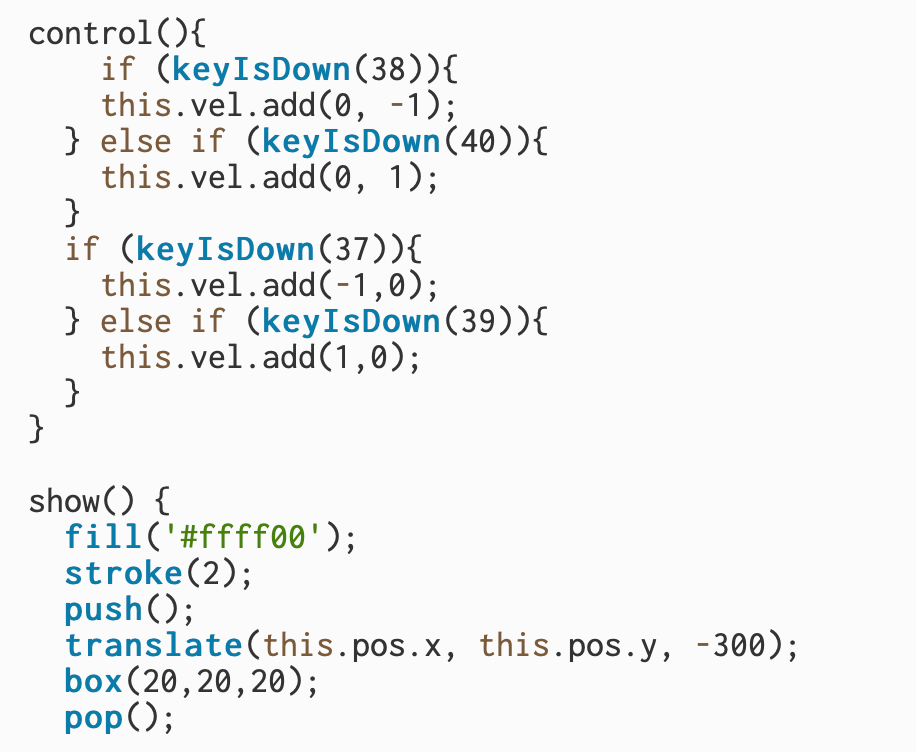
The player object also contains methods for movement control (according to the arrow key being pressed, I add to the velocity element to generate motion).
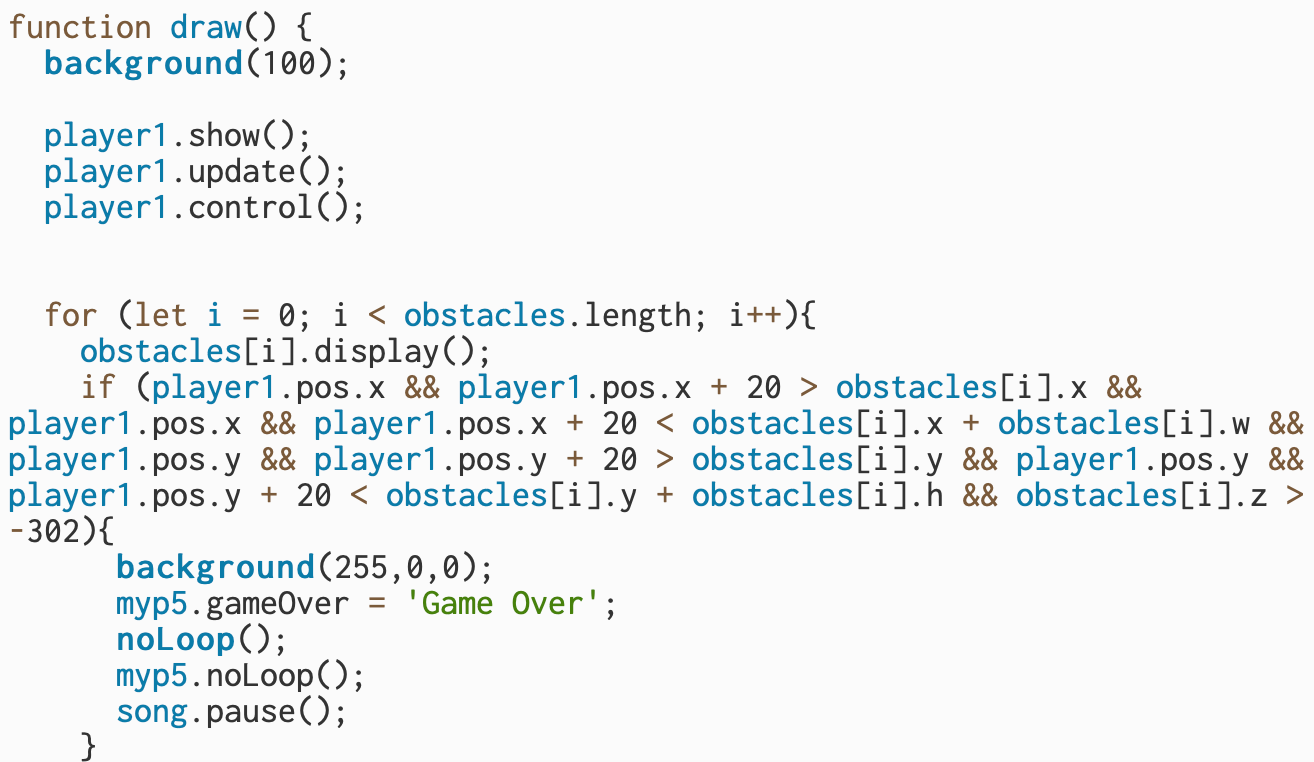
This was the most difficult part of the project; figuring out how to create a 3D object collision detection method. Ultimately the method works as follows: if the player object's x and y positions are within the box's dimensions, and the box's z position is past the z position of the camera, then stop the sketch.
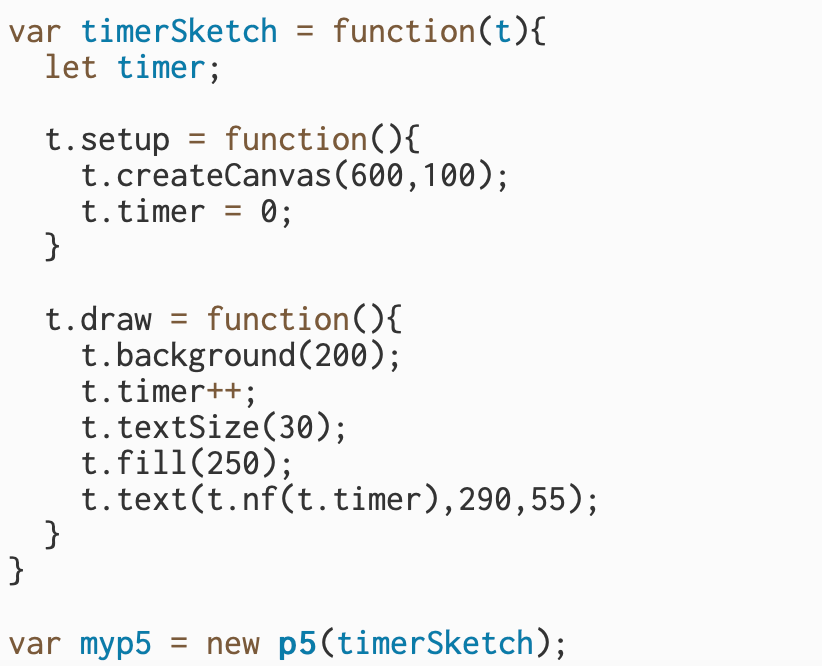
The separate timer sketch.
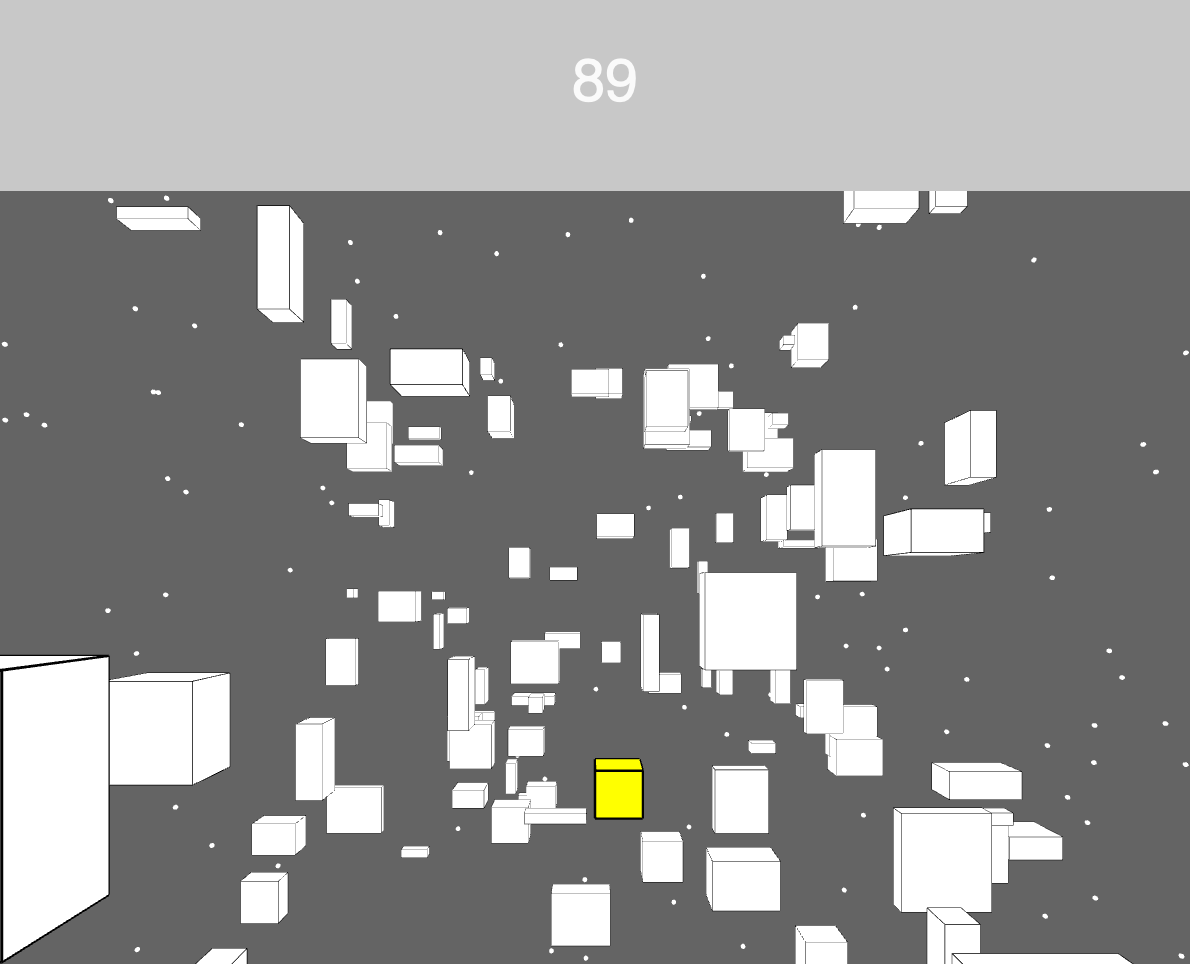
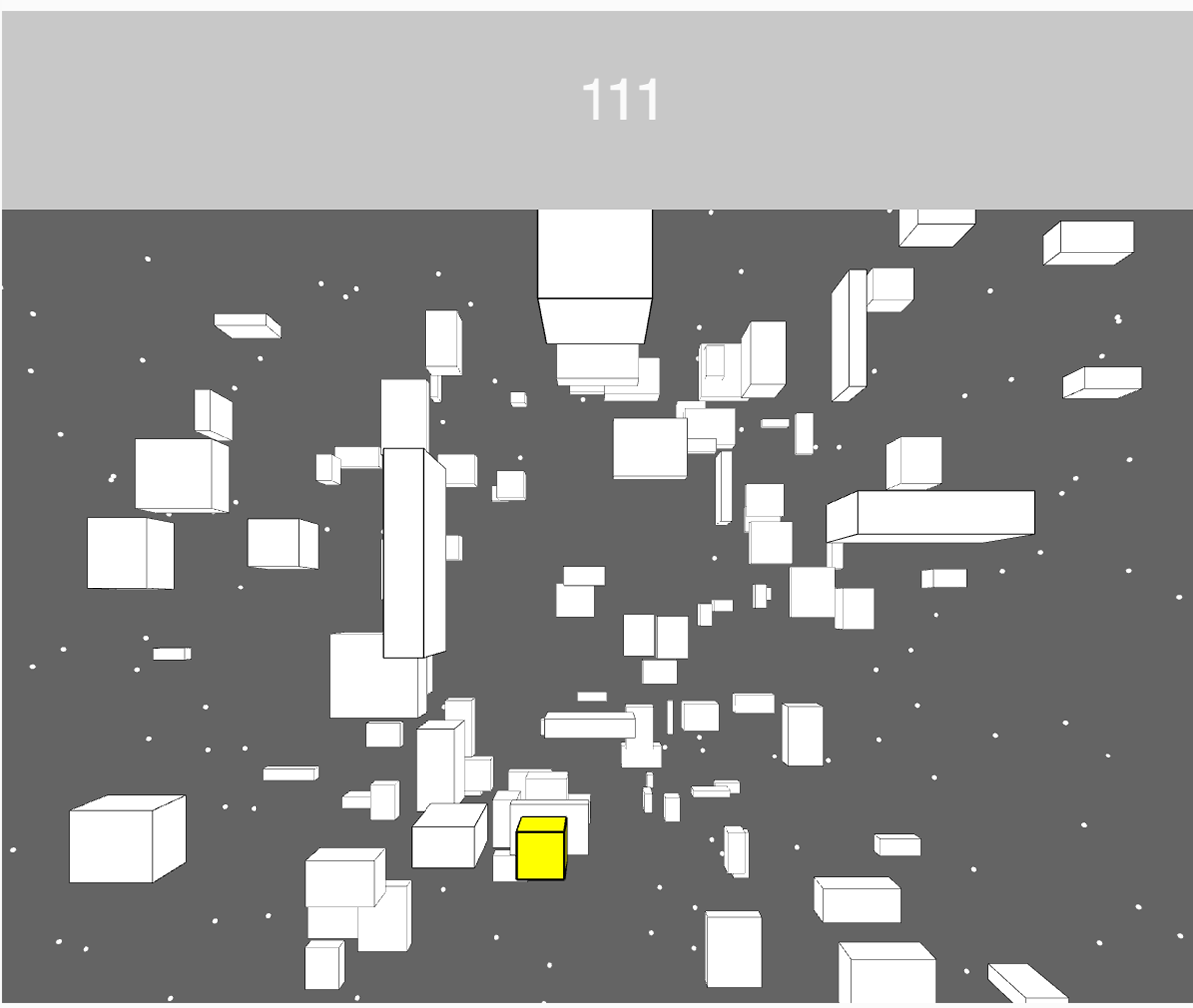
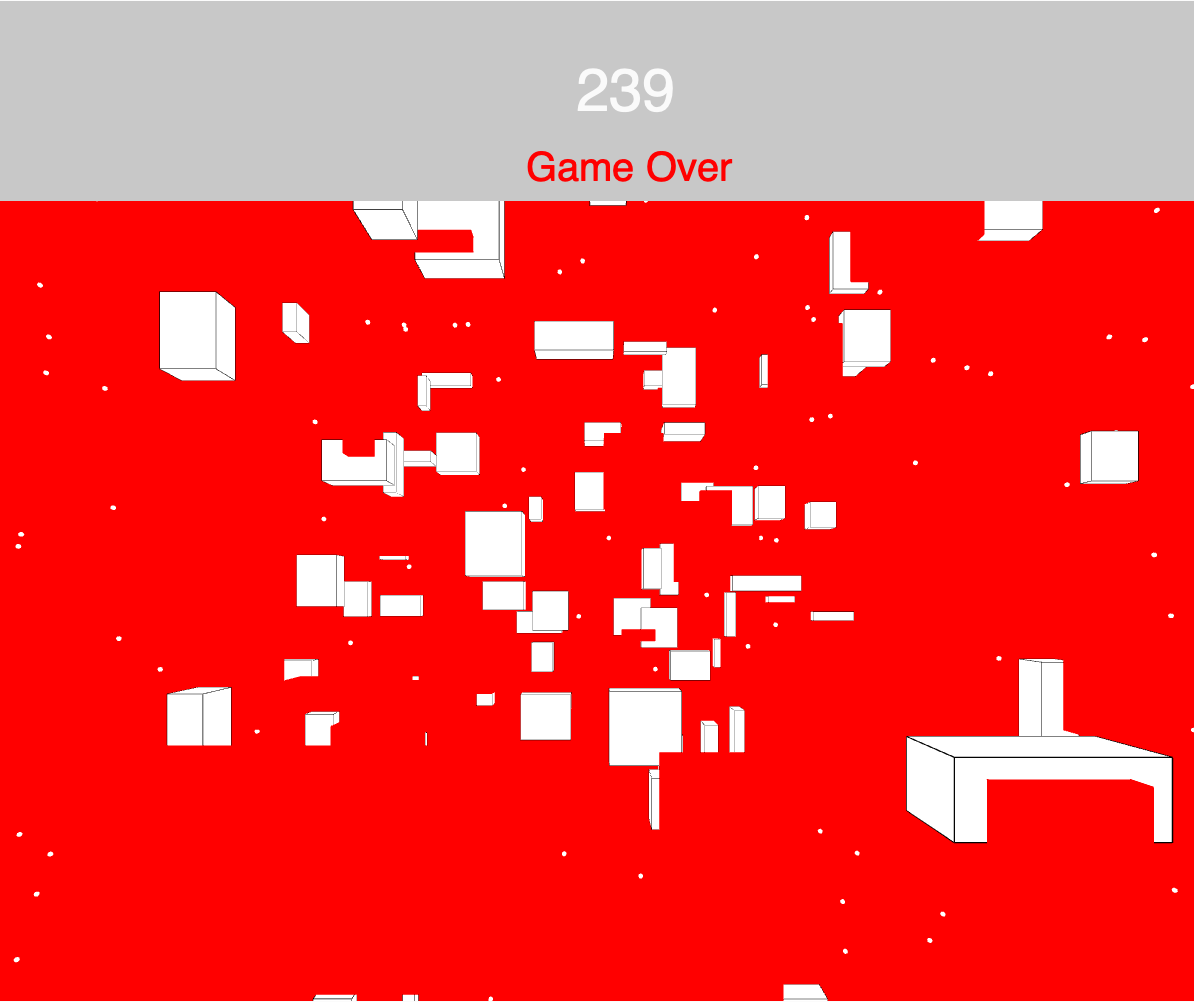